If you want an introduction to Feature Flags – what they are and why you should use them, check out my previous post, here. In that post, we also got a step-by-step tutorial on how to setup feature flags using Launch Darkly, a turnkey Feature Management service, in a Vue application. Today, let’s look at Azure App Configuration – a service provided by Microsoft, tackling the same problem. If you’re already committed to the Azure ecosystem, App Configuration could make sense as you can turn to the familiar Azure portal and provision an instance of this service, just as you would do for any of the hundreds of other offerings there. Just like in the previous episode, I’ll walk you through how to provision and configure an App Configuration instance and then show you how to utilize it from within a Vue application.
Setup Your Azure App Configuration Instance
Head over to the Azure Portal and search for App Configuration. Let’s provision a new instance by providing the relevant details. This entails providing a name for your instance, the geographical region where you want this resource to be provisioned and the pricing tier that you want to go with. The service comes in two pricing tiers – a free edition and a standard edition. That’s pretty much it and is usually provisioned in a few minutes.
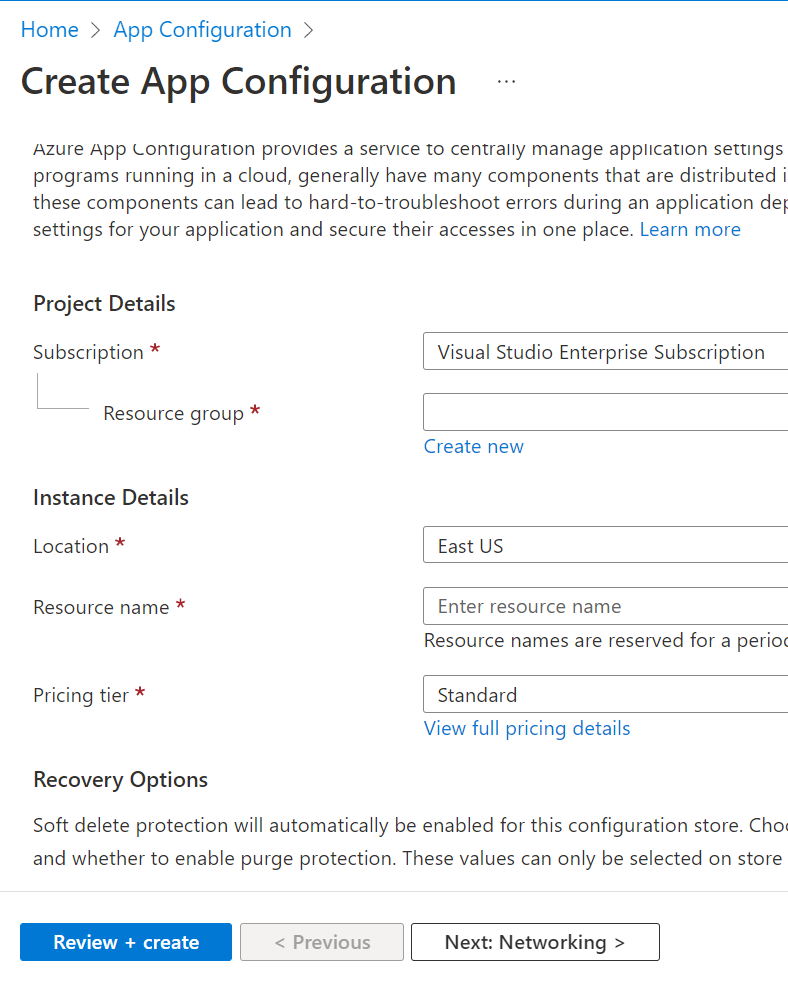
Once your instance is created, navigate to the access keys section and you’ll have access to a few secrets associated with your instance. Grab the connection string from the “Read-only keys” tab. You’ll need this to later connect to this instance from a Vue application. As a client-side JavaScript app won’t be able to store any secrets securely, we’ll want to rely on the read-only connection string to simply grab feature-flag values from the server.
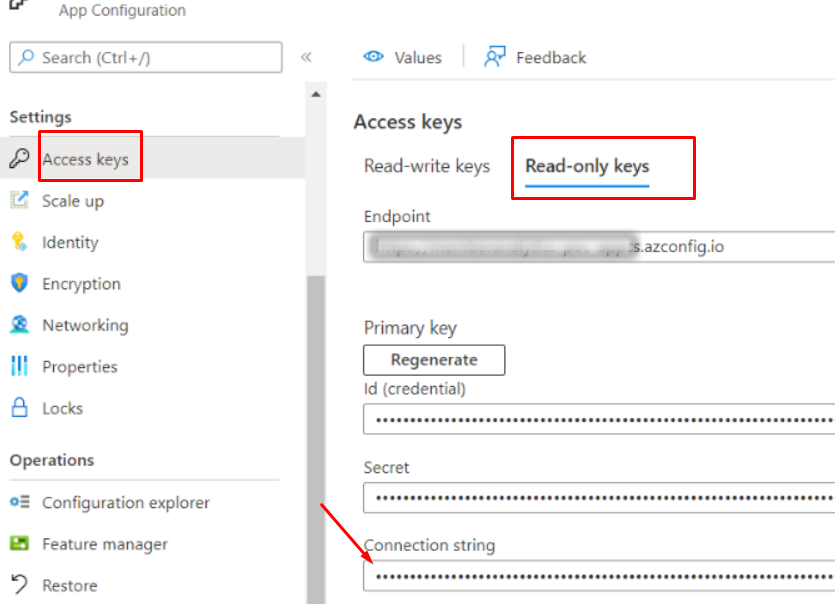
Next, head over to the “Feature Manager” section and create one or more feature flags. At a minimum, you’ll want to supply a unique name for each feature flag you create. Optionally, you can also provide a label. One way to utilize these labels is to use them as environment designations. For instance, if your application is hosted in a QA environment and you have a separate Production environment, assign a label to each feature flag you create so that you can independently control them in each of your environments.
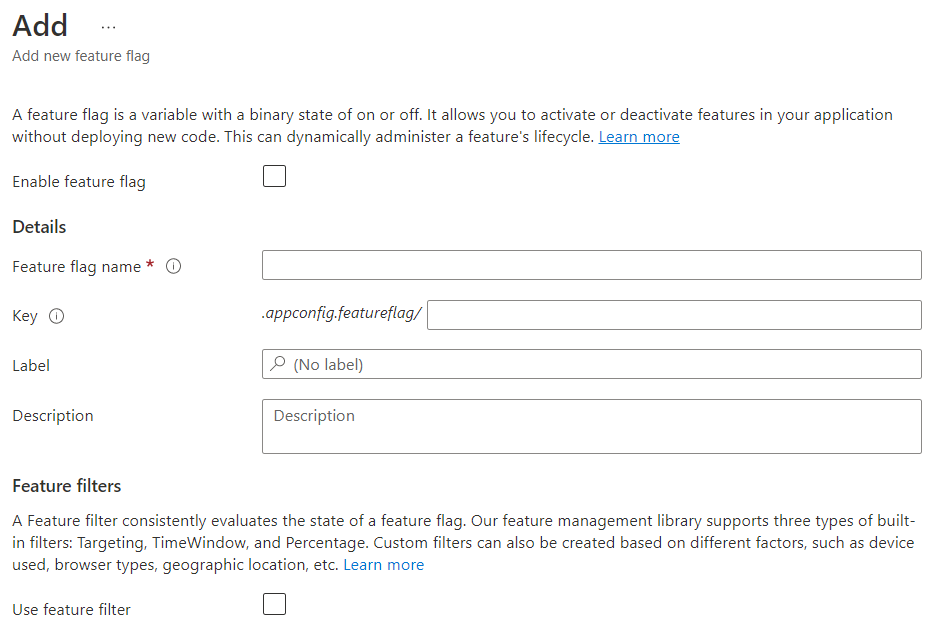
For instance, you may want feature X to be enabled in QA while you may want to disable it in Production. Labels provide you a method to set up something like that. Alternatively, you can have separate Azure App Configuration instances for each of your environments, as well.
Using Azure App Configuration Feature Flags in Vue
Let’s test this out using in a Vue 3 application. I have bootstrapped a brand new Vue app using the create-vue tool. We’ll install two npm packages from Microsoft to connect to our Azure App Configuration instance from our Vue application:
npm install @azure/app-configuration @azure/identity
Next, let’s create a plugin in Vue, as a helper class, where we can tuck away the plumbing code that we’ll write to connect to Azure and pull our feature flags while providing neat and simple access to all our components that needs access to these flags. You can learn more about Vue plugins by reviewing the docs. Below is the plugin that I wrote. It provides a neat helper method called getFeatureFlag
where a caller can get a feature flag value by simply providing the key (the unique name) of the feature flag.
import { AppConfigurationClient } from '@azure/app-configuration'; const connectionString = 'you-azure-configuration-readonly-connection-string-goes-here'; let client = null; if (connectionString) { client = new AppConfigurationClient(connectionString); } const featurFlagsManager = { async getFeatureFlag(name) { try { const response = await client.getConfigurationSetting({ key: `.appconfig.featureflag/${name}`, label: 'local' // you can use an environment variable to inject this at runtime, if necessary }); return JSON.parse(response.value).enabled; } catch (error) { console.error('error in feature flag fetch', error); return false; } } }; export default { install: (Vue, options) => { // inject a globally available property Vue.provide('$featurFlagsManager', featurFlagsManager); } };
Now, you can wire up this plugin in your main.js along with the bootstrapping of the rest of your Vue application.
import { createApp } from 'vue' import App from './App.vue' import azureAppConfigPlugin from '../azureAppConfigPlugin'; const app = createApp(App) app.use(azureAppConfigPlugin); app.mount('#app');
Now, you can inject the $featurFlagsManager
in any of your components and ask for a particular feature flag. Check out my component below that looks for a sprinkles
feature flag, and based on its current value, displays either an ice cream cone with sprinkles or a plain one instead.
<template> <div> <h1>Hello Azure App Configuration Feature Flags</h1> <pre>{{ flags }}</pre> <div v-if="flags['sprinkles']"> <img src="@/assets/sprinkles.jpg" width="300" height="400" /> </div> <div v-else> <img src="@/assets/plain.jpg" width="300" height="400" /> </div> </div> </template> <script> import { inject } from "vue"; export default { async mounted() { const $flagsManager = inject("$featurFlagsManager"); this.flags.sprinkles= await $flagsManager.getFeatureFlag('sprinkles'); }, data() { return { flags: { sprinkles: true, }, }; }, }; </script>
Closing Remarks
You can find a working copy of the example above in my GitHub repo, here:
In my brief experience with this service, I have noticed that there is some caching that is being employed behind the scenes. You may not receive an updated feature flag value on the very next request that you make with the JavaScript client… it may be a few seconds or minutes before the updated value is returned in the response.