You may have a piece of code that you want to execute on a scheduled basis. Maybe you want to run some calculations on your Salesforce org every night and post the results to an API in one of your other systems. Maybe you want to check a remote system for a status update on something and inform your Salesforce org of that update. Thankfully, such constructs exist within Salesforce. However, in my personal experience, some applications of those constructs are simpler than others. For instance, if you have something that you want to run once a day, you can do so using a setup screen built into Salesforce whereas running the same job once every hour would require you to schedule that through some additional Apex code. Let’s look at some examples.
For this demonstration, I have created a Custom Object named Log Entry, as shown below:
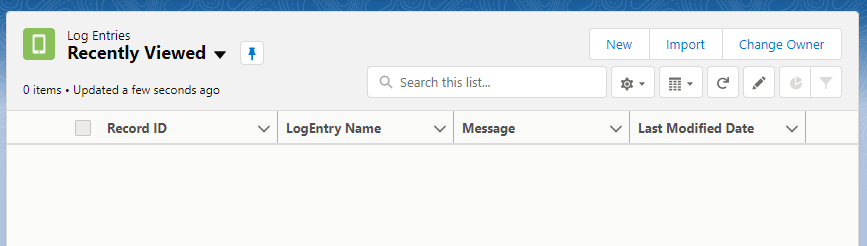
I also created a new Apex class with a single static method to pump some data into this example object. I marked the method as a @future method as I want this piece of code to run in a separate thread. I’ve also marked it callout=true to show that this method could be reaching out to a third-party API (although this example is not doing that). The test method simply inserts a new record into our LogEntry custom object.
public class LogEntryGenerator { @future (callout=true) public static void generateLogEntry() { // This is a method where we'll pretend we are calling a remote API and then creating a record in an object based on that. LogEntry__c test = new LogEntry__c(); Datetime myDT = Datetime.now(); String myDate = myDT.format('**yyyy-MM-dd HH:mm:ss**'); test.Message__c = 'Entered at ' + myDate; insert test; } }
Next, in order to run this on a schedule, we’re going to be calling this from another Apex class and method that implements the Schedulable interface in Apex. This interface requires a single static method called execute. You could have just as easily written the code that you want to run on a scheduled basis within that execute method but it’s probably best to follow my pattern here, to contain your business logic in a separate class altogether. Such a pattern allows you to mark the method as a future method, to specify that it makes a callout and such and it probably also helps in readability as the business need is encapsulated in one construct while the Salesforce construct of “scheduling code” can reside in another place.
global class ScheduledLogEntry implements Schedulable { global void execute(SchedulableContext SC) { LogEntryGenerator.generateLogEntry(); } }
Apex Scheduler
Our work is not done, just yet. We need to instruct the Salesforce system to now execute our code on a schedule that we like. We can do so by navigating to the Apex Scheduler.
From Setup, search for “Apex” and select “Apex Classes” and click on the “Schedule Apex” button.
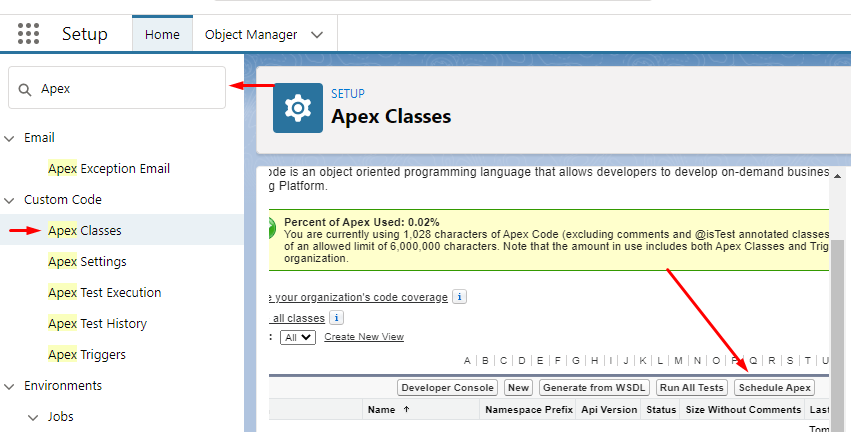
Using this entry screen, you can specify a name for your scheduled job, specify what days you want it to run and a preferred start time:
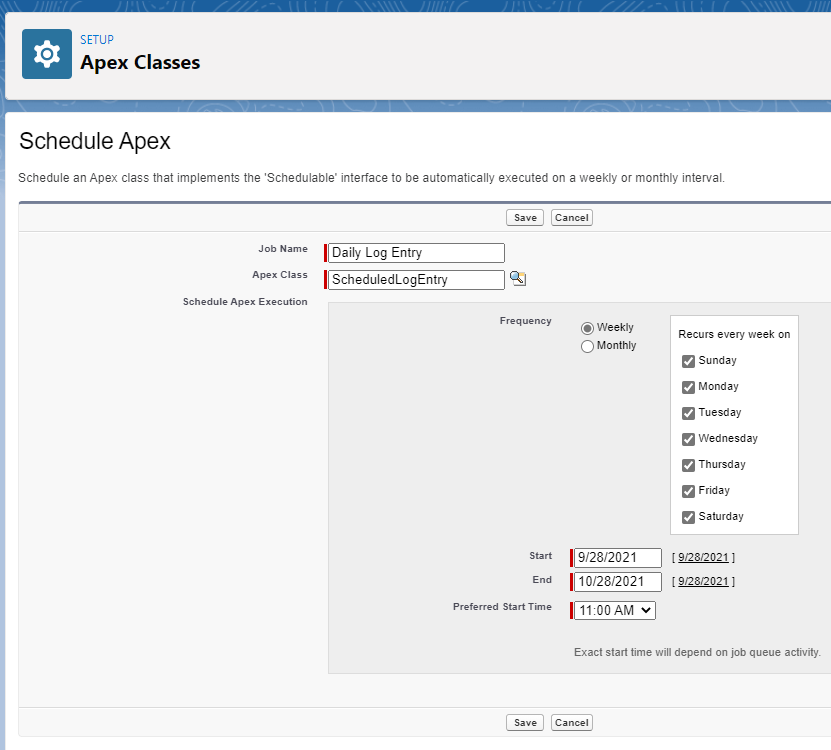
Scheduling via Code
But, wait… what if you want to run this more frequently than once a day? What if I want to run this once every hour? You’ll have to write an additional snippet and execute them from the “Execute Anonymous Apex” window from within the Developer Console:

Above, I’m using the System.schedule method to create an Apex schedule, programmatically. I’ve named my job “Daily Log Entry”. The second parameter may look strange to you if you are unfamiliar with Cron, a system utility that comes with Unix based systems, used for scheduling jobs. That strange looking string is called a “Cron expression” and in my particular example, I’m specifying that my job should run once every hour, at the top of the hour.
View / Delete Jobs
Once you create these scheduled jobs, you can get a listing of them by searching for and selecting “Scheduled Jobs” from within Setup. You can also delete a job, here.
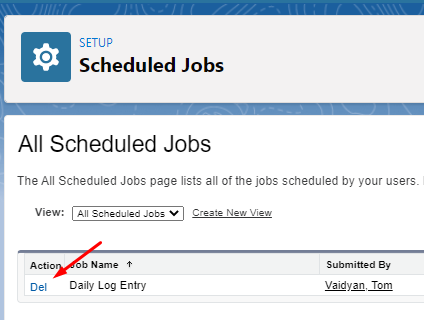
Parting Thoughts
You can learn more about Cron from its associated Wikipedia article. However, keep in mind that not all Cron implementations and expressions are supported by Salesforce. For instance, if you want to schedule something to run every ten minutes, you’ll need to repeat the above expression 6 times with different minute settings, effectively creating six different schedules. Also, keep in mind that there are many limitations imposed by Salesforce about scheduled apex code. Read the docs carefully, to avoid running into implementation issues.