If you’re collaborating on a C# / .NET development project with someone else, whether that is just another person or a team of several developers, consider using EditorConfig. If you’re unfamiliar with this project, you can check them out at editorconfig.org. In short, you use an .editorconfig
file to define stylistic rules of your source code – tabs vs spaces? variable naming rules, braces or no braces, var or explicit types, etc.; you can set the severity of the rule to be a mere suggestion to a concrete error that causes the build to fail if the rule is not adhered to (side note: yes, you can use silent to denote that the rule should be ignored). You commit this file along with the rest of your source code so that other team members have access to these rules and use their own IDEs and code editors to enforce these rules on their local copies of the source code.
But Why?
- It helps maintain a consistent style in your codebase. Such consistency will help make your codebase more approachable. There is less cognitive load to content with as patterns will emerge out of your codebase from one file, to another.
- It helps new members of the team. Especially, if that new team member is new to programming altogether, EditorConfig will help them to adhere to your naming conventions and styles. In my experience, new programmers are not pouring our boring standards documents of the language prior to writing their code.
- It helps team members in reading each other’s code faster, allows them to jump in and help troubleshoot each other’s issues. It helps make pair-programming sessions go smoother.
- It’s quite possible, especially for .NET development these days, that you and your team members are on different operating systems, IDEs and code editors, all working on the same project. Your IDE or code editor may have its own way of formatting code. If this formatting is not consistent across the team, when it comes time to committing your code, your editor may complain (or even auto format) your team members code in the styles that you have set. If this happens, you’re faced with either ignoring those other changes that your editor made and then committing your actual changes or commit those other changes causing others to scratch their heads as to what you changed in their files. This could result in unnecessary frustration and friction among your team members. A tool like EditorConfig will help avoid these issues by setting up a consistent style for your codebase, across your team.
Using EditorConfig in Visual Studio
All recent versions of Visual Studio have built-in support for .editorconfig files. That is, you don’t have to download or install anything extra to use .editorconfig files in Visual Studio. It will automatically take note of any .editorconfig files in the project/solution that you have open and enforce those rules, whether by displaying suggestions, warning, or errors denoting any discrepancies from the prescribed styles. And Visual Studio doesn’t stop there – if you open a .editorconfig file (which is just a text-based file that you can open with any text-editor), it will give you a neat UI, segmenting your rules into different categories, checkbox and dropdown controls to specify your selections and a search widget! Did you expect anything less from Visual Studio? But, if you want to see the raw text file, as is, you can always right-click the file in your Solution Explorer and choose “Open With” and select the “Source Code (Text Editor)” option.
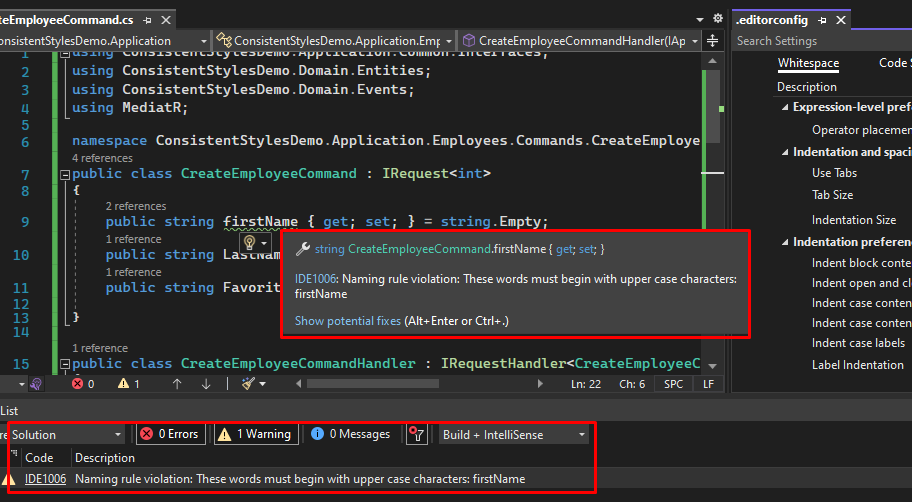
Using EditorConfig in VSCode
The best way to integrate EditorConfig into your workflow on VSCode is to first use the C# extension by Microsoft (powered by OmniSharp). Make sure that this extension is enabled.
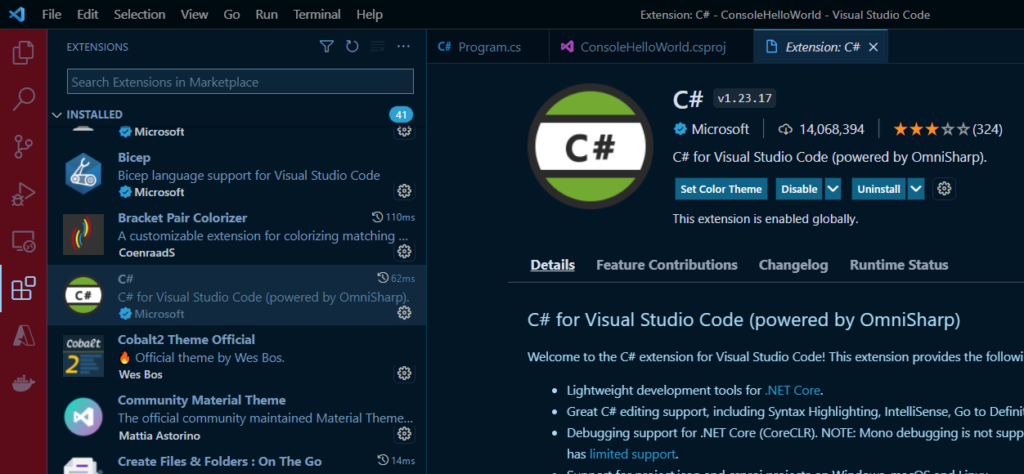
Next, create an omnisharp.json
file at the root of your solution/project folder with the following contents:
{ "RoslynExtensionsOptions": { "enableAnalyzersSupport": true }, "FormattingOptions": { "enableEditorConfigSupport": true } }
With that in place, VSCode (and more specifically the C# extension) will recognize any .editorconfig files in your project and start giving you notifications on any code style issues that it encounters.
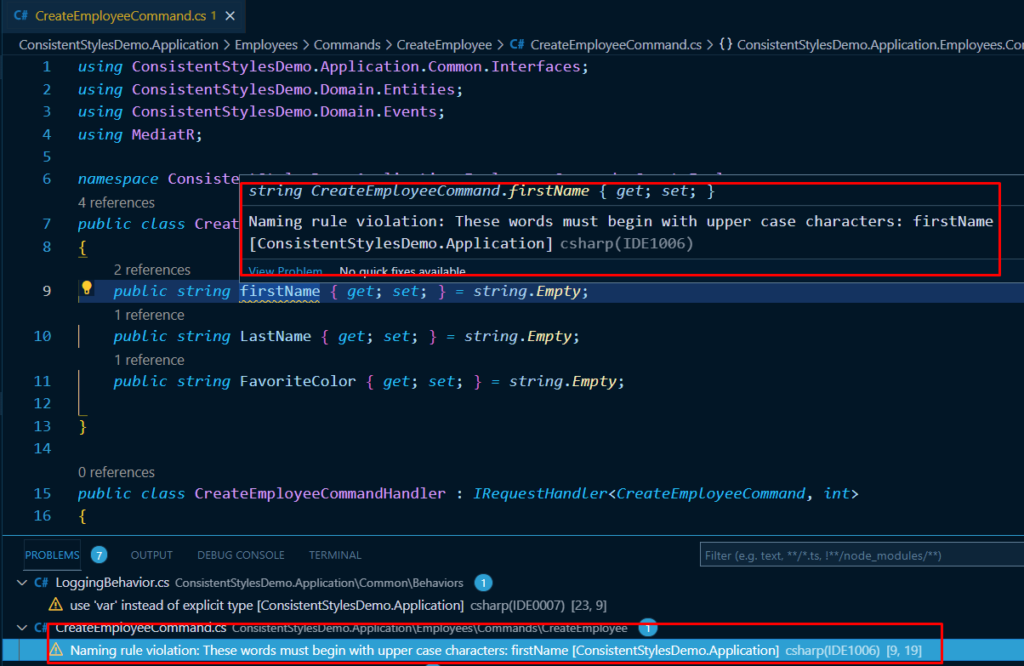
Parting Thoughts
Hopefully, I have convinced you of the usefulness of EditorConfig. You may be a senior developer or a team lead, wanting to implement this in your team and in your codebases. Where do you start? Where can I get a list of all the C# specific rules that I can use in a .editorconfig file? You can start by checking out the .NET Code Style Rule Options documentation page on Microsoft Docs. In addition to giving you a good overview and links to other related docs, this page gives you a good example .editorconfig file that you can use as a starting point for your own projects. However, personally, I’d recommend changing the severity of some of those naming rules from a mere “suggestion” to a “warning” or “error” so that they are a bit more noticeable by the team.
Also, keep in mind that the EditorConfig initiative is not directly related to C# and .NET. You can use the same approach for keeping your JavaScript, Python, C, C++ and other codebases stylistically consistent.