If you have been working with .NET technologies for more than five minutes, you have surely come across Newtownsoft Json.NET. It is the most downloaded package on NuGet. It can do everything that you can hope for and dream of doing with JSON data in your dotnet apps. It is a time-tested, thorough, extremely feature-rich JSON library. There is a ton of documentation around it – both official and community-driven. Aside from that, you can probably find a code-snippet that matches your exact JSON parsing need on Stack Overflow.
And that’s precisely the reason why I wanted to write this post.
There you are trying to quickly complete a Jira ticket that’s been assigned to you. You need to deal with some JSON. Perhaps you’re calling a third-party API from within your .NET app that’s returning you some JSON. Maybe there is a JSON string that’s tucked away in a database table somewhere that you need to hydrate into a CLR object. You google your symptoms, and it inevitably leads you to a blog article or a Stack Overflow post that tells you to:
Step 1) Download Json.NET
Step 2) Solve your problem
There’s nothing inherently wrong with that approach. But I just merely wanted to point out that there is a noteworthy JSON parser built-in, natively within the dotnet framework. This was not always the case and that’s the void that Json.NET so perfectly filled for so many years. But if you are new to the dotnet world and haven’t already built that muscle memory with Newtonsoft Json.NET and/or your needs are rather straightforward and the only reason why you were springing for that library was because you didn’t know that you had other options, you may want to give the built in System.Text.Json
offering a whirl.
System.Text.Json
System.Text.Json was released as part of .NET Core 3.0 which came in September 2019. So, it’s been around for a few years now but is still largely eclipsed by Json.NET’s gargantuan legacy. Prior to this release, ASP.NET itself used the Newtonsoft Json.NET Library under the hood for JSON serialization and deserialization. With the introduction of System.Text.Json, that work is now delegated to the built-in counterparts.
Let’s look at a few examples.
Serialization Examples
Below’s the ubiquitous Employee demo class. Let’s hydrate it and serialize it into a JSON string.
using System.Text.Json; internal class Program { private static void Main(string[] args) { Console.WriteLine("Hello, World!"); var employee = new Employee { EmployeeId = "123", FirstName = "Tom", LastName = "Vaidyan", Email = "tom@test.com", Manager = new Employee { FirstName = "John", LastName = "Smith", Email = "jsmith@test.com" } }; var employeeJson = JsonSerializer.Serialize(employee); Console.WriteLine(employeeJson); } } public class Employee { public string FirstName { get; set; } = string.Empty; public string LastName { get; set; } = string.Empty; public string EmployeeId { get; set; } = string.Empty; public string Email { get; set; } = string.Empty; public Employee? Manager { get; set; } }
Running that will produce the following JSON string output:
{"FirstName":"Tom","LastName":"Vaidyan","EmployeeId":"123","Email":"tom@test.com","Manager":{"FirstName":"John","LastName":"Smith","EmployeeId":"","Email":"jsmith@test.com","Manager":null}}
That’s not prettified for human-readability. If you want that, you can pass in a JsonSerializerOptions
object in your serialize call, like so:
var employeeJson = JsonSerializer.Serialize(employee, new JsonSerializerOptions { WriteIndented = true });
That’ll produce output that looks like this:
{ "FirstName": "Tom", "LastName": "Vaidyan", "EmployeeId": "123", "Email": "tom@test.com", "Manager": { "FirstName": "John", "LastName": "Smith", "EmployeeId": "", "Email": "jsmith@test.com", "Manager": null } }
Although that’s syntactically correct, semantically, you’ll want to use camelCase when outputting JSON. You can set that within the JsonSerializerOptions
object.
var employeeJson = JsonSerializer.Serialize(employee, new JsonSerializerOptions { WriteIndented = true, PropertyNamingPolicy = JsonNamingPolicy.CamelCase });
That’ll give you output, like so:
{ "firstName": "Tom", "lastName": "Vaidyan", "employeeId": "123", "email": "tom@test.com", "manager": { "firstName": "John", "lastName": "Smith", "employeeId": "", "email": "jsmith@test.com", "manager": null } }
Deserialization Examples
You can just as easily take a JSON payload and hand it off to this library and it will hydrate objects for you. Take the JSON output from earlier and use it to call the Deserialize
method.
var jsonString = "{\"FirstName\":\"Tom\",\"LastName\":\"Vaidyan\",\"EmployeeId\":\"123\",\"Email\":\"tom@test.com\",\"Manager\":{\"FirstName\":\"John\",\"LastName\":\"Smith\",\"EmployeeId\":\"\",\"Email\":\"jsmith@test.com\",\"Manager\":null}}"; var result = JsonSerializer.Deserialize<Employee>(jsonString);
And without much ado, it will create the object(s) in C# for you.
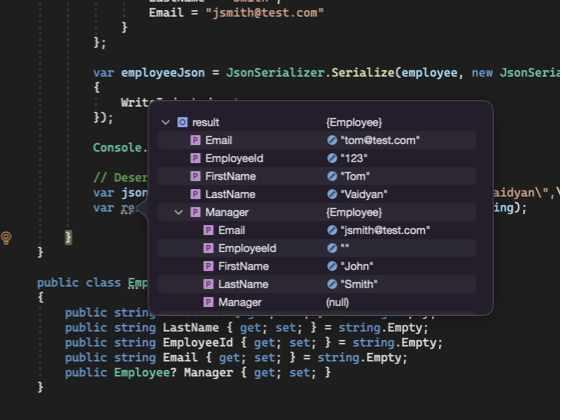
If you have a property in your JSON structure that doesn’t match exactly in name with your C# property, you can help the parser by decorating that property with a JsonPropertyName
attribute.
[JsonPropertyName("empId")] public int EmployeeId { get; set; }
Closing Remarks
There you have it – basic JSON scenarios covered in System.Text.Json eliminating the need for you to bring in another library. Checkout the docs for all the deets.
You may come across benchmarks showing you that System.Text.Json is so much more performant than Json.NET. Don’t switch solely because of such claims. Nine times out of ten, the negligible performance gains mean nothing to you and your use-case and provides no discernable difference in your applications. Instead, go with the tools that better fit your needs – the ones with better ergonomics (the ones that you find easier to use), with the features that you really need, and ultimately – the ones that make you happy.
You can find the code from today’s episode on my GitHub account, here: